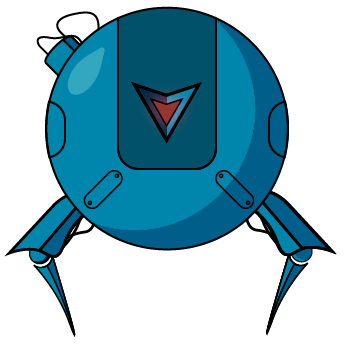
[](https://travis-ci.org/rubenlagus/TelegramBots)
[](https://jitpack.io/#rubenlagus/TelegramBots)
[](https://addo37.github.io/AbilityBots/)
[](https://telegram.me/JavaBotsApi)
[](https://ghit.me/repo/rubenlagus/TelegramBots)
Usage
-----
**Maven**
```xml